A Quick Tour of Java Data Types
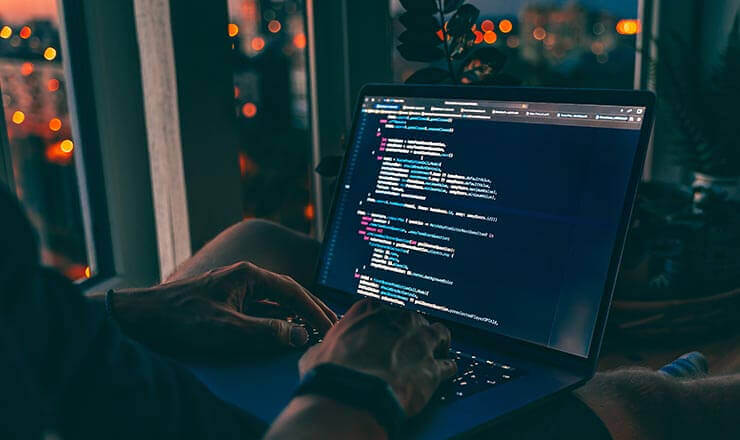
In programming, data types are classification of units of data that determine how the programmer can manipulate them and what type of mathematical, relational or logical operations can be performed on them. In this article, we’ll take a quick look at some of the most common data types in the Java programming language.
Integer Data Types
byte: 8-bit signed two’s complement integer
short: 16-bit signed two’s complement integer
int: 32-bit signed two’s complement integer
long: 64-bit signed two’s complement integer
The standard Java integer data types are byte, short, int, and long.
These data types represent signed integers of varying sizes. The byte and short data types are 8-bit and 16-bit signed integers, respectively.
The int data type is a 32-bit signed integer, while the long data type is a 64-bit signed integer.
These data types are used to store numeric values in Java programs.
As with all data types, the size of an integer value is determined by its data type. For example, an int value takes up four times as much space as a byte value.
When choosing an integer data type for a particular value, you should consider both the range of values that can be represented and the amount of space that is available.
In general, you should use the byte or short data types for small values that will fit within their respective ranges, and the int or long data types for larger values.
Floating-Point Data Types
float: 32-bit IEEE 754 single-precision floating point number
double: 64-bit IEEE 754 double-precision floating point number
Float and double are two of the most commonly used data types in programming.
As their name suggests, they are used to represent real numbers with fractional parts.
Float is a single-precision data type, which means that it can represent numbers with up to 7 digits of precision.
Double is a double-precision data type, which means that it can represent numbers with up to 15 digits of precision.
While float offers less precision than double, it is often the preferred data type for real-world applications due to its smaller memory footprint.
Boolean Data Type
boolean: true or false value
In computer science, the Boolean data type is a data type that can have only two possible values (usually denoted as True and False), intended to represent the two truth values of logic and Boolean algebra.
In most programming languages, these are intended to be used as truth values, though some languages treat them as numeric values (1 for True, 0 for False).
The Boolean data type is named after George Boole, who first defined an algebraic system of logic in the mid 19th century.
The Boolean data type is primarily used to store boolean values (true or false), but can also be used to store binary data.
For example, the number 12 can be represented as 111100 in binary, which is stored in six bits.
When storing boolean values in binary form, each value is stored in a single bit.
In some cases, such as when storing large numbers of boolean values, it can be more efficient to use a format known as packed Bools, which packs multiple boolean values into a single byte or word.
This can reduce the amount of memory needed to store the values, and can also reduce the time needed to access the values.
Character Data Type
char: 16-bit Unicode character
The char data type in Java is a single 16-bit Unicode character.
It has a minimum value of ‘\u0000’ (or 0) and a maximum value of ‘\uffff’ (or 65,535 inclusive).
Char values are used in representing unicode characters and because of this, they are often referred to as “unicode characters”.
In java, chars are represented by the class java.lang.Character.
The char data type is an unsigned primitive data type meaning that it can hold positive or negative values.
Although the char data type is a signed primitive data type, its range of values is 0 to 2^16 – 1.
Char elements are declared using single quotes (‘ ‘). A good example of a char literal is ‘a’. Java also supports escape sequences for special characters such as ‘\t’ which represents a tab character.
When a char is declared with a value out-of-range, such as -1, it will be stored as its corresponding unsigned value, which in this case would be 2^16 – 1 or 65,535.
The Char data type is ideal for storing characters that need to be processed individually such as digits in mathematical operations or for storing keystrokes entered by the user. Char literals are enclosed in single quotes (‘ ‘), for example: ‘A’, ‘$’, and ‘\u0041’ ( Unicode character 41).
A Char can also be expressed as an integer between 0 and 65535 using the following notation: (char)intValue; where intValue is any valid integer between 0 and 65535.
See: How to input Char values?
How to output Char values?
What is the size of Char?
What’s the range of Char?
Can a Char variable contain more than one character?
Is Char a primitive or derived data type?
What’s the difference between Char and String?
What’s the difference between ASCII and Unicode?
How do I convert from one character encoding to another?
What’s the relationship between chars and bytes?
For example, to store the letter A you could write: (char)65 . When using the Char data type it is important to remember that it only stores one character not multiple characters.
If you need to store multiple characters then you should use the String data type instead. The String class provides methods for handling Strings of text such as concatenation, comparison, searching, and modification.
As you can see, there are a variety of data types available in Java. The type you use will depend on the specific needs of your program.Hopefully this article has given you a helpful overview of some of the most common data types used in Java programming.